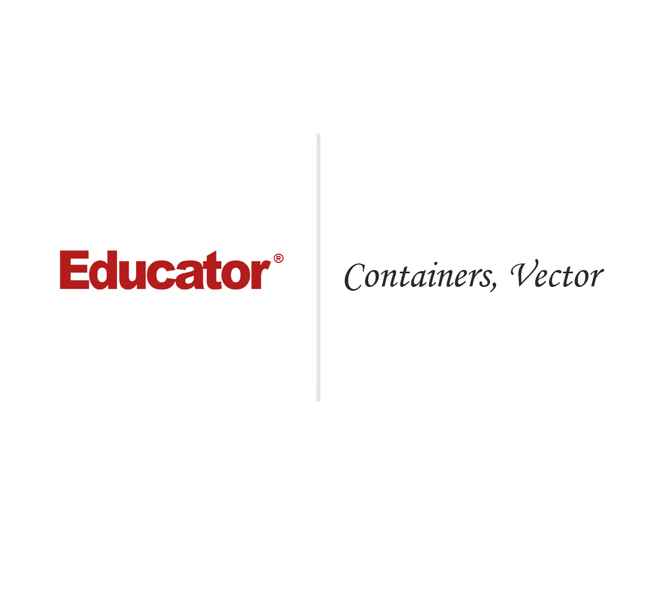
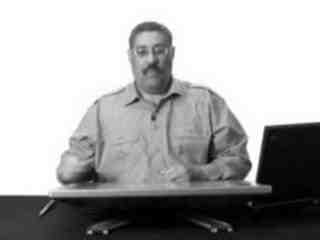
Alvin Sylvain
Containers, Vector
Slide Duration:Table of Contents
Section 1: Intermediate C++
Overview of Intermediate C++
39m 28s
- Intro0:00
- Overview0:22
- Review3:10
- Language Features You're Expected to Already be Familiar With3:41
- Introduce New Features and Cover Some Familiar Territory More In-Depth4:17
- Containers; Vector4:45
- Features: Templates and Containers4:56
- Vector is the First Container Learned5:15
- Example5:44
- Containers; List7:18
- Linked List is One of the Most Basic Data Structures in Computer Science7:24
- This Class Shows How to Use the Standard Library 'List' Container7:52
- Example8:15
- Containers; Set, Map9:15
- Binary Tree9:23
- 'Set' and 'Map' are Not Sequential10:23
- Set Retrieves Unique Keys10:41
- Map Retrieves a Unique Key, Then the Related Value10:55
- Example11:11
- Templates12:00
- Allow for Code Re-Use and Modularity12:14
- Example12:46
- Object Oriented Programming15:19
- OOP is the Main Motivating Factor to the Original Development15:29
- Only Grafted on Non-OOP Features15:39
- Discuss How Classes and Objects Are Created and Instantiated in C++16:20
- Operator Overloading18:29
- Writing Polymorphic Functions18:39
- Operator Overloading is a Standard Feature in Most Programming Language18:52
- Example19:00
- Exception21:05
- Practical Programming Means Handling Unexpected Conditions in an Expected Way21:08
- Programmers Have Used Different Techniques to Pass Along Info That's 'Less Than Good'22:27
- Exception Handling in C++23:51
- C++ Exceptions Can be Any Type24:31
- Example24:38
- Namespaces25:30
- Name Collision25:40
- C++ Solution is for Each Company to Use Their Own Unique Namespace26:06
- Example26:29
- Input/Output27:43
- Class Hierarchy for C++ Input/Output28:16
- Turn on Exception Handling and Check Input/Output Status Bits28:21
- How to Use 'Stringstream' Object28:32
- More Details for Functions29:19
- Parameters on the Main Function29:34
- Inline Functions29:52
- Recursive Functions30:34
- Pass Pointers to Functions as Function Parameters30:42
- More Kinds of Types32:23
- Union to Force Memory Overlap32:30
- Enum Type33:01
- Typedef Keyword33:37
- Keeping Things the Same 'Const'34:00
- Pre-Compiler Macros34:09
- '#include'34:22
- '#define'34:45
- Conditional Compilation with '#ifdef'35:19
- Compiling the Code35:57
- Dash D Testing36:26
- Common Flags37:18
- Flag That Allows a Run-Time Debugger to Hook Into the Application38:11
- Tally Ho!39:07
Review
56m 23s
- Intro0:00
- Overview0:21
- Acquire a Compiler1:24
- Windows1:51
- Macintosh2:02
- Acquire a Compiler for Linux2:18
- Redhat2:28
- Ubuntu2:38
- Software Manager2:51
- Acquire an Editor3:14
- Popular Editors3:48
- Compile and Run a Program5:00
- Traditional First Program5:28
- Comments in Code8:12
- Standard C 'block' Type Comment8:34
- C++ 'to end of line' Comment8:59
- Simple Data Types10:00
- Integer10:07
- Floating Point11:11
- Character11:50
- Two Types of 'Strings' in C++12:31
- Examples12:48
- Operators15:56
- Unary16:01
- Binary17:24
- Ternary18:16
- Function-like18:43
- Member Reference19:41
- Expressions20:51
- Modifying21:01
- Arithmetic21:24
- Comparison21:46
- Logical22:24
- Comma22:48
- Left-Hand Expressions24:04
- 'Right hand'24:07
- 'Left hand'24:30
- Examples25:12
- 'If' Branching26:33
- 'Switch' Branching29:15
- Looping30:59
- 'While' Loop31:04
- 'Do While' Loop32:17
- 'For' Loop32:59
- 'Break'34:15
- 'Continue'34:36
- Functions34:49
- Prototypes36:06
- Function Parameters36:36
- Return Value38:23
- Overloading38:41
- Arrays39:10
- Multi-Dimensional Arrays41:05
- Pointers43:37
- Definition43:43
- 'Dereference' Operator43:51
- 'Reference' Operator44:40
- Structures47:20
- Example: Without Structure48:12
- Example: With Structure49:13
- Object Oriented Programming51:00
- Access Can Be Defined As53:24
- Input / Output54:07
- Insertion Operator54:29
- Extraction Operator55:04
- File Stream Objects55:33
- Two Thumbs Up!55:58
Containers, Vector
40m 14s
- Intro0:00
- Lesson Overview0:11
- Containers1:50
- Vector1:58
- List2:24
- Set, Map2:56
- Data Access3:44
- Iterators4:10
- Container Information4:37
- Vector4:53
- Memory Re-Allocation6:07
- Declare Vector of 'Type'7:37
- Size & Capacity8:01
- Vector<type> Variable8:20
- Vector<type> Variable (Initial Size, Initial Value)9:27
- Vector<type> Variable (Initial Size)10:11
- Access Vector Data10:27
- Example: Typical Array Access10:46
- Method Call Access11:07
- Other Data Access12:26
- Front12:30
- Back12:38
- Use Empty Function to Test13:37
- Vector Information14:36
- Resize15:53
- Reserve17:55
- Vector Iterators19:11
- Example: Data Elements May be Accessed Like Standard Array19:30
- Data Elements May be Accessed Using Iterators20:38
- Vector Iterator Begin/ End23:30
- Begin23:34
- Eng23:50
- Reverse Begin24:07
- Reverse Iterator24:16
- 'Short?' Cuts25:21
- Declaring Loop Index Inside the 'for' Loop25:34
- With Iterators26:05
- Modifying Vector Data26:54
- Push Back27:44
- Pop Back28:57
- Insertions29:32
- Example: Insert New Value at Beginning29:51
- Example: Insert Value in Middle30:40
- More Vector Operations32:12
- Empty32:15
- Erase32:22
- Clear33:01
- Assign33:20
- Compile-Time Initialization34:34
- Example 135:22
- Example 236:07
- Example 337:25
- Caution-Invalidating Iterators37:59
- Contained!39:56
Containers, List
33m 40s
- Intro0:00
- Lesson Overview0:13
- Linked List Overview1:34
- Arrays and Vectors1:39
- Linked List3:16
- List Information5:53
- Empty Method6:04
- Max Size6:14
- Resize6:51
- List Access7:48
- Front and Back8:04
- Iterators8:16
- Example8:49
- List Iterators vs. Vector Iterators9:24
- The Next Element May be Physically in Memory Before the Current Element9:50
- Vector Iterators Allow Integer Add10:34
- List Iterators Do Not Allow Integer Add12:24
- Simple List Modification14:24
- Constructors14:32
- Difference Between Assign and Insert15:16
- Assign15:37
- Insert16:01
- Push16:31
- Pop16:38
- Erase16:53
- Complex List Modification18:34
- Merging and Splicing18:38
- Merge19:35
- Splice20:24
- Reordering21:46
- Removing22:28
- Modification Examples23:22
- Functions as Parameters24:49
- A Function for 'remove_it()'25:04
- A Function for Merge and Sort - Evens Before Odds25:46
- Deque27:28
- Insertion or Removal in Middle May be More Efficient than with Vectors27:51
- Not Guaranteed to be Contiguous Memory28:42
- Same Method as Vector, Plus More29:00
- Stack29:11
- Empty, Size, Top, Push, Pop31:05
- Queue31:56
- Definition32:19
- Not That Kind of List33:19
Containers, Set, Map
35m 35s
- Intro0:00
- Lesson Overview0:11
- Tree Structures for Sets/Maps2:01
- What's the Value?3:00
- Pair Template Class4:45
- Allows a Function to Return 'Two' Values Instead of the Normal One5:15
- Example for an Integer Set5:46
- Example for Map with a String Key, Double Value7:09
- Set, Multiset8:03
- What's a Set8:32
- Elements are the Key9:30
- Custom Class for Ordering9:52
- Get Data In to Set10:28
- Constructors10:33
- Insert (Value)11:26
- Insert (Position, Value)12:30
- Insert (First, Last)13:18
- Get Data Out of Set13:28
- Erase13:33
- Find13:57
- Lower_Bound15:20
- Upper_Bound15:43
- Iterators Positioned Such That a For-Loop Can 'Start at Zero'15:59
- Set Examples16:32
- Map, Multimap19:15
- Get Data In to Map20:45
- Constructors20:48
- Insert a Key Value Pair21:25
- Insert Position22:05
- Insert (First, Last)22:21
- Get Data Out of Map22:37
- Operator23:43
- Erase25:16
- Find, Lower & Upper Bound25:28
- Map Examples25:37
- Comparison Class29:34
- Example30:37
- What About Duplicates?33:29
- Define a Pair to Receive a Container Iterator and a Boolean33:40
- Example34:07
- Useful Maps35:10
Templates
41m 8s
- Intro0:00
- Lesson Overview0:08
- Function Template5:48
- Define5:52
- Use6:46
- Example7:03
- Constraints on Types10:34
- Example10:45
- Multiple Types13:58
- Example14:03
- Default Types17:11
- Example17:24
- Class Template20:27
- Example20:33
- Template Specialization24:31
- Example25:00
- Non-Type Parameters27:32
- Example27:46
- Templates in Templates32:20
- Example32:34
- Cautions35:04
- Don't Use a Template with a Type That Doesn't Support All the Template's Operations35:10
- Templates Can Not be Split into Header Files and Compiled Units36:51
- Issues with Debugging39:47
- Templates Gone Wild!40:33
Object Oriented Programming, Part 1
1h 4m 48s
- Intro0:00
- Lesson Overview0:14
- Concepts4:39
- Historically Distinct Ways of Programming and Design4:41
- OOP Emphasizes Important Concepts6:28
- Some Terminology9:53
- Object9:56
- Class11:47
- Instance12:56
- Example14:04
- Abstraction20:00
- Abstraction Defines the Essential Characteristics of an Object20:08
- Emphasis on Essential20:34
- Classes are Defined with Characteristics That Distinguish One Class of Objects From Another21:18
- Encapsulation22:23
- Private Data23:25
- Public Methods23:40
- Protected Data24:47
- Inheritance25:52
- What Is Inheritance?25:58
- Example27:57
- Polymorphism29:18
- Example of Operator Overloading29:30
- Example of Parameter Signature30:22
- Methods Inherited From another Object Can be Overwritten and Customized to the New Object31:13
- Object Relationships33:37
- Consider Two Objects, Car and Vehicle33:42
- Consider Two Other Objects, Car and Tire34:01
- Object Motorcycle34:22
- Inheritance Example35:27
- Multiple Inheritance39:24
- Example39:51
- Be Careful for Diamond Problem43:46
- Multiple Inheritance Example45:28
- Constructors46:55
- Default Constructor47:33
- Copy Constructor49:10
- Default Constructor50:33
- Example50:55
- Shallow Copy52:41
- Example53:10
- Deep Copy56:35
- Example56:42
- Copy Constructor1:01:34
- Good Design? Or…1:04:28
Object Oriented Programming, Part 2
44m 16s
- Intro0:00
- Lesson Overview0:10
- Overloading Constructors3:10
- Provide Other Constructors3:26
- Example3:43
- Initialization Lists5:40
- Syntax Example5:43
- Equivalent to Initializing in Statements in the Method Body6:50
- Destructors7:09
- Destructor is Automatically Called when Instance is Deleted7:26
- Declare the Declare as Virtual in the Event the Class is Designed to be Derived by Sub-Class9:15
- What's in a Name?11:15
- Example: How to Tell Field from Parameter11:56
- Friendship Functions16:32
- Avoids Strict Object Oriented Principles and Declare the Function or Class Using 'friend' Keyword16:47
- Example17:12
- Friendship Classes18:48
- Example18:57
- Designing for Derivation20:54
- Add 'Virtual' Keyword to Public Methods21:50
- Add 'Virtual' Keyword to Destructors24:17
- Abstract Class25:41
- Designed Only for Derivation25:47
- Example26:13
- Compilation Units28:22
- Classes Are Typically Divided into the Interface Portion and Implementation Portion28:53
- Namespaces Are Used to Associate a Class Method with the Class Definition29:31
- Example Compilation Units30:53
- Definition for 'Vehicle' Class for an Include File30:54
- Implementation for 'Vehicle' Class in Program File33:01
- Why You Add 'Include Guards'36:33
- Suppose You Derive 'Automobile' From 'Vehicle'36:38
- Caller File Includes Both37:21
- Object Oriented? Or Not?38:46
- When Is It Appropriate?39:31
- When Is It Not Appropriate?41:04
- The Object Oriented Purist43:53
Operator Overloading
1h 12m 54s
- Intro0:00
- Overview0:13
- When Not to Overload Operators4:32
- Arithmetic Operators Should Only Apply to 'Numeric' Kinds of Classes4:53
- Complex Number6:04
- 'add' Operation vs. 'add()' Method6:38
- When It Can Be a Good Idea7:42
- Your Class is Inherently Numeric In Nature7:49
- Some Operators Still Make Sense to be Overloaded8:26
- Kinds of Operators to Overload11:03
- Binary Operator11:19
- Unary Operator12:06
- Non-Member Functions14:27
- Example: Named Vector15:03
- Named Vector is a Directional Vector with a Name15:10
- Directional Vectors15:15
- Overloading Demonstration18:58
- Assignment Operator=20:26
- Deep Copy20:37
- Copy Constructor20:55
- Overloading Operator=22:21
- Example Member Function22:28
- Overloading Binary Operator==27:10
- Example 'Equal' Member Method27:30
- Other Comparison Methods Can Call This One31:09
- Overloading Binary Operator<32:30
- Example: 'Less Than' Member Method32:43
- Overloading Arithmetic Operators35:04
- '@=' Operator35:23
- Operations Work Symmetrically36:22
- Overloading Binary Object-to-Object38:19
- '@=' Is Member Function with Full Access38:27
- '@' is Non-Member Function40:24
- Multiple a Vector by a Number to Make it Larger43:19
- Need Two Symmetrical Non-Member Functions44:46
- Overloading Unary Operator47:16
- No Parameter for the Operator@ Function47:40
- Overloading Prefix/Postfix Operator49:12
- Unary Operators with Different Semantics for Prefix and Postfix49:38
- '++' and '--'50:43
- Overloading Prefix Operator++51:21
- Add 1.0 to X/Y Components Before Accessing Value for Caller51:38
- Also Include the Operator-- to Subtract 1.051:55
- Overloading Postfix Operator++52:02
- Give It a Dummy Operator52:07
- Overloading, Object-to-Other Object54:18
- Make Non-Member Function a 'Friend' to Access Private Members of Your Class54:36
- Parameters Must Include Other Objects When Overloading54:50
- Useful for Saving an Object to a File55:52
- Overloading Operator<<56:45
- Include Non-Member 'Friend' Function in Class56:50
- Implement Function in the Compilation Unit57:58
- Allows Output of Your Objects with Other Data59:39
- Array Operator[]1:00:55
- Example: The Standard Library Vector1:02:00
- Named Vector1:02:49
- Overload Array Operator []1:03:19
- Implement Operator[] As a Member Function1:03:23
- Write the Code1:04:15
- It Lets You Define a Non-Int for the 'Array Index'1:05:22
- Function Operator()1:06:39
- Examples1:07:07
- Define (idx)1:08:38
- Overloading Function Operator()1:09:45
- Implement Operator() As a Member Function1:09:48
- Calling Example1:11:27
- Bad Overloading!1:12:27
Exception Handling
57m 51s
- Intro0:00
- Overview0:11
- Something Went Wrong! Now What?6:05
- Data Must Always be Validated6:11
- Many Operations Will Validate Input to Make Sure No Mistakes Were Made8:13
- Many Check the External Environment10:26
- Many Don’t Check But App Can Still Crash10:56
- Different Error Checking Methods11:38
- Traditional Method11:42
- Exception Handlin14:09
- Lazy Way15:30
- Traditional Error Checking16:08
- Example: Return Code, Simply 'if'16:12
- Example: Output Parameter18:15
- Exception Handling20:01
- 'Try' Block20:05
- 'Catch All' Block20:49
- No 'Finally' Block21:31
- 'System Errors'22:34
- Basic Syntax23:12
- Code to Throw an Exception23:16
- Code to Handle an Exception23:49
- Example Exception26:51
- Run This Program Fragment, It Will Crash26:54
- Run This One, The Error is Controlled28:00
- Throw Exception30:51
- Can 'Throw' An Exception If Error Conditions Found30:56
- Basic Example' 'int' Exceptions31:03
- Character String Exceptions36:16
- Exception Can Be Any Type, But a Character String Can be Informative in Simple Cases36:19
- Not Good for More Than Simple Situations38:19
- Derive From 'Exception' Class38:34
- When You Don’t Care Exactly Which Sub-Class of 'Exception' Can Be Thrown39:02
- When Each Sub-Class Handled Differently39:17
- Exception Derivation Example41:28
- Adding 'Throw()' Keyword to Prototype45:32
- Promises to Compiler What Kind of Exceptions the Function or Method Might Throw45:42
- Leave the Keyword Off46:39
- Leave the Parameter List Empty46:51
- Exception Can Not Be Caught With a Type-Specific Handler47:28
- Exceptions Not Derived From 'Exception'49:11
- Don’t Rely on System Exceptions!54:06
- Certain System Operations Don't Throw an Exception in a Platform-Independent Manner54:10
- Examples54:25
- Your Own Code Should Test for the Conditions That Might Lead to Uncatchable Exceptions56:03
- Exceptional!57:30
Namespaces
29m 51s
- Intro0:00
- Overview0:15
- Namespaces5:27
- Uses the 'Scope Operator'5:30
- Two Kinds: Standard Library and Classname5:47
- Helps Avoid Third Party Name Collision6:42
- 'Namespace' for Class Association7:46
- Scope7:53
- Example8:01
- Implementation in Separate File, Same Scope8:46
- Define a Namespace10:05
- Use 'namespace' Keyword, Valid Identifier, and Block to Add to a Namespace10:07
- Example10:40
- Units Can Share Namespace12:26
- 'std' Namespace12:40
- Include Namespace Declaration Block in Each Compilation Unit13:13
- Use Namespaces for Types, Classes14:04
- Compilation Unit: alpha.cpp14:11
- Compilation Unit: beta.cpp14:27
- Calling Unit14:49
- Don't Use Namespaces for Instances15:21
- Namespace Cannot Be Used to Duplicate Names of Object Instances in Different Compilation Units15:25
- Example15:40
- Error When Compilation Units Are Linked Together16:22
- Using Explicit Namespace16:51
- Header File for Class16:56
- Use of Namespace in Compilation Unit17:03
- 'Using' Keyword17:36
- Specify a Default Namespace17:40
- More Than One 'Using' Can Be Specified18:49
- Avoiding 'Using'20:48
- Third-Party Packages Often Avoid 'Using'20:49
- Example21:03
- Using for Particular Names22:54
- The 'Using' Keyword Can Set Up Just Certain Names for a Default Use22:56
- Naming Strategy24:30
- Unique Name, World-Wide24:48
- Avoid Confusion with Another Similar Name25:23
- Typical Advice: Use Company Website Name25:30
- Namespace Aliases28:35
- User Uses Certain Namespace with a Different Name28:27
- Example28:38
- Avoiding Duplicates29:33
Input/ Output
42m 46s
- Intro0:00
- Overview0:11
- Streams4:37
- Byte Streams4:51
- Operator '<<' Overloaded for Output5:14
- Operator '>>' Overloaded for Input5:47
- I/O Class Hierarchy6:46
- ios_base6:57
- Input Stream7:04
- Output Stream8:27
- Multiply Inherits From istream, ostream9:04
- Buffer Class Hierarchy9:27
- Buffering Allows I/O to Work More Efficiently9:38
- 'Flushed'9:54
- Streambuf - Buffer for Data9:58
- Base Class Attributes10:25
- Formatting Information10:36
- State Information14:43
- Sub-Class Members15:46
- IOS Adds Additional Members and Methods15:49
- Error State Flags18:23
- Input Classes20:31
- istream - Input Character Stream20:33
- ifstream - Input File Character Stream22:50
- istringstream - Input String Character Stream23:23
- Output Classes24:10
- ostream - Output Character Stream24:13
- ofstream - Output File Character Stream25:28
- ostringstream - String Character Stream25:42
- Multiple Inheritance and Includes25:59
- iostream - Multiply Inherits From istream, ostream26:04
- Classic Example of Multiple Inheritance26:22
- fstream - Inherits From iostream26:47
- stringstream - Inherits From iostream27:08
- Detect Failures Using Status Bits27:32
- Test the Status Bits27:43
- Use Exceptions29:12
- Failure Bit Cautions30:20
- failbit Is Set on Formatted Input if the Input Stream Cannot be Parsed30:27
- Example30:37
- String I/O with Stringstream32:21
- Used for Input and Output32:26
- Example33:45
- Flushing Data buffer35:39
- Sometimes Buffering Is Not Desired35:43
- Input Flush Example37:10
- Output Flush Example38:17
- Internationalization39:06
- Complex Problem with Different Cultures39:20
- 'Imbue' I/O with Installed Locale Info40:12
- Set Size of a 'Character' for I/O41:45
- Confused Yet?42:47
More With Functions
42m 52s
- Intro0:00
- Overview0:18
- Parameters for 'Main'2:18
- First Function2:19
- The Way Seen Most Often5:13
- Some Typical Parameters6:05
- Help6:11
- Version6:22
- User6:51
- Password / Port6:59
- File To Be Operated On7:25
- Code Example8:25
- Some Things of Note12:17
- First Item in 'argv' is the Program File12:20
- How Each Argument is Given to the Program is OS Dependent13:42
- A Return of '0' Usually Means Success14:48
- More Things of Note15:11
- It's Customary to Have Both Stand-Alone Arguments (Options) and Arguments with Additional Parameters15:28
- Examples15:37
- Inline Functions17:37
- 'inline' Tells the Compiler to Insert the Function Body Explicitly Into the Code17:43
- Function Modularity Without Function Overhead17:53
- Example19:05
- Recursive Functions20:22
- Definition of Recursive: A Function or Algorithm That Uses Itself to Solve a Problem20:34
- Common Mistakes20:56
- Classic Example: Factorial21:27
- Recursive Factorial Code23:16
- Compare Iterative Version23:48
- Pointers to Functions25:55
- Technique Used Often in GUI Programming26:05
- The Package Calls the Caller's Function by Dereferencing the Pointer26:45
- Example27:04
- Pointers to Functions as Parameters27:45
- Syntax28:01
- Calling Parameter Function Within Function29:42
- Example30:15
- Multi-Dimensional Array Parameters31:07
- Function Receives a Pointer to Array But Can Not Know Size Ingo31:40
- Example31:48
- Ways to Handle; 1-Dim Array33:53
- Good use for an Inline Function34:06
- Ways to Handle: Internal Data35:38
- Example 135:48
- Example 236:25
- Ways to Handle: Objects39:38
- Example39:47
- Usage Examples41:18
- No Help Here!42:28
More Types
39m 10s
- Intro0:00
- Overview0:12
- More Kinds of C++ Types1:32
- It Has Strong Type Conventions That Can Be Used to Enforce Program Integrity1:50
- Recommended: Avoid the Short Cuts!2:02
- If a Short Cut is Necessary…2:57
- Unions3:24
- Union = Short-Cut3:25
- Example: Inspect Each Byte of a 32 bit Integer3:56
- This May Not Run the Same on Different Platforms5:11
- Union of Struct8:12
- Typical Use for a Union is Sharing Memory Between Structures8:13
- Example8:21
- Union Rules9:56
- There's No Platform Independent Way to Store Member Data9:57
- Cannot Store Classes That Have Constructors11:37
- Enumerators12:39
- Useful for Defining Enumerated Constants12:40
- Use it Like Any Other Type13:02
- Example: Can Be Started and Restarted at Any Value13:38
- Can Be Used Like Any Integer14:48
- Less Error-Prone15:10
- Be Careful Changing the Sequence15:25
- Example: Sometimes Useful to Associate String Constants16:24
- Use of 'typedef'17:46
- In C, 'types' Created with 'typedef' Keyword17:47
- In C++, the Keyword is Largely Unnecessary17:52
- Examples: Use of'typedef' in C and C++18:06
- Custom Type Substitution19:57
- 'typedef' Keyword is Often used in Place of Somet Other underlying Type for Purposes of Clarity19:58
- Examples: Custom Type Substitution20:25
- Pointer Hiding23:10
- Pointer to a Type Look23:11
- Example: Pointer Hiding23:39
- Cautions Using Underlying Types26:02
- Example: Using Underlying Types26:03
- The Underlying on Most Systems Today26:42
- Example: Only Works Because It Is an 'int'27:20
- Cautions Using time_t Typedef28:05
- 32-Bit Integer 'Runs Out' In The Year 203828:06
- Only Use the Provided Classes and Functions Designed to Handle a Particular Type29:27
- When Working with typedef, Treat It Like A Class Object29:45
- 'const' Ness30:45
- It’s More Than Just Declaring a Variable or Parameter That Cannot Be Changed30:46
- Also Used on Methods31:25
- Example: 'const' ness31:37
- 'const' on Object Attributes33:01
- Can Be Used on Object Attributes33:01
- Example: 'const' on Object Attributes33:06
- Row That Is Permanently Identified by 1733:34
- Allow Certain Change, 'mutable'34:29
- 'mutable' Allows Change34:30
- Used on Specific Object Members When the Method Is Supposed to be 'const'34:49
- Why Would Some Members Need to Change?35:23
- Examples36:00
- 'mutable' Examples36:49
- Union Troubles38:53
Macros & Compilation
51m 23s
- Intro0:00
- Overview0:14
- Pre-Processor Macros2:57
- Define with the 'Pound' Character2:58
- Macros Are Pre-Processed on a Textual Basis3:48
- Including Other Files5:25
- Use the 'Include' Macro When You Use a Pre-Compiled Library5:26
- Triangle Brackets6:20
- Quotes Indicate That the Include File May Be Found in the Same Directory as the File Being Compiled6:32
- Any Text File Can Be Included7:00
- Basic Constant Definition8:54
- 'Define' Macro Keyboard8:55
- 'MAX_ROWS replaced by '300'10:16
- Compiler Never Sees MAX_ROW10:48
- Literally Anything Can Be Defined12:03
- Typical Use12:19
- Application Constants12:30
- C++ Improvements12:48
- Using Macros in Macros13:32
- Macro Substitution Can Occur in Macro Substitution13:33
- Example14:17
- Text Replacement14:30
- Conditional Compilation, Includes16:20
- ifdef, ifndef, if, else, elif, and endif16:21
- Include Guards & Example18:10
- Conditional Compilation20:21
- Don't Print Out Debugging Info in the Production Code20:22
- Compiler Will Never See the Testing Code21:48
- Macros Can Be 'undef'ed22:36
- Good for Temporary Removal23:27
- 'Comment Out' Sections23:37
- Can't Use the // or /**/ Comment23:54
- Use 'ifdef'24:57
- Macro Functions26:29
- Macros Have a Facility for Creating Small In-Text Functions26:30
- Define a Macro Function27:16
- If You Need More Than One Line27:32
- Macro Function Examples28:34
- Return Area of a Circle28:35
- Return Volume of a Cylinder29:00
- What Can Happen Without Parentheses?29:45
- Avoid Those Problems31:14
- In C++, Use Inline Functions31:15
- Inline Functions Avoid All Other Problems That Historically Have Occurred With Macros32:11
- Mixing C with C++34:08
- C Code Can Not Be Linked Directly with C++ Code34:09
- In C Header File Add35:38
- Pre-Defined Macros37:18
- Macros That Should Be Pre-Defined for Every Standards-Compliant Compiler37:19
- Occasional Misuse38:39
- Abusing the Facility38:40
- Example: Trying to 'look' Like Pascal39:03
- Allows Code to be Written Like This39:20
- Compiling40:14
- Typical Compilation Command Line40:15
- Compilation of One Unit at a Time41:02
- Compiler Flags to Find Libraries42:34
- Finding Other Include Files & Other Libraries to Link Into Your Application42:35
- Example: Create an Application that Uses the MySQL Database43:02
- Other Useful Flags47:07
- Small Set of Command Line is Most Common47:08
- '-c' Create an Object File From the Source47:12
- '-g' Add Internal Code That Allows a Run-Time Debugger to Hook into the Program47:22
- '-Dmacro' Set a Macro to be Defined47:58
- '-E' Stop After Pre-Processing48:55
- '-S' Stop After Compilation49:26
- Setting Flags for IDE49:51
- If You're Using Eclipse49:52
- Example50:25
- Uh, Almost51:03
A Look at Some Code
57m 58s
- Intro0:00
- Real Code Example0:29
- Global Constants1:10
- Account.cpp2:14
- Account.h3:03
- Call Back Function3:24
- Vector & Transactions4:10
- Constructor5:54
- User.h8:54
- User.cpp12:01
- Exceptions23:56
- Class Exception, Minor Exception, and Regular Exception24:57
- StringField.h29:38
- StringField.cpp35:40
- NumberField.h38:56
- NumberField.cpp44:56
- IntegerField.cpp47:53
- Date.h49:17
- Date.cpp51:10
- BankAccountMain.cpp52:20
- Lesson Summary56:46
Loading...
This is a quick preview of the lesson. For full access, please Log In or Sign up.
For more information, please see full course syllabus of Intermediate C++
For more information, please see full course syllabus of Intermediate C++
Intermediate C++ Containers, Vector
Lecture Description
In this lesson, our instructor Alvin Sylvain goes through an introduction on containers: vector. He starts by explaining containers and vectors. He then discusses the different types of vectors to declare, accessing vector data, vector information, iterators, modifying vector data, insertions, vector operations, compile-time initialization, and caution-invalidating iterators.
Bookmark & Share
Embed
Share this knowledge with your friends!
Copy & Paste this embed code into your website’s HTML
Please ensure that your website editor is in text mode when you paste the code.(In Wordpress, the mode button is on the top right corner.)
×
- - Allow users to view the embedded video in full-size.
Next Lecture
Previous Lecture
1 answer
Last reply by: Ram Manohar Oruganti
Tue Nov 4, 2014 6:27 PM
Post by Ram Manohar Oruganti on November 4, 2014
Hi,
I thought that begin and end give the value of at the start and end. So, how come the iterator could know the address when given a value?
1 answer
Tue Nov 20, 2012 7:20 PM
Post by Edwin Clement on November 17, 2012
How can I write a function maximum that computes the largest element in a list<int>